Demonstration of the Available Metrics#
For a complete list of metrics and their documentation, please see the API Metrics documentation.
This demonstration will rely on the results produced in the "How To" notebook and serves as an extension of the API documentation to show what the results will look like depending on what inputs are provided.
A Jupyter notebook of this tutorial can be run from
examples/metrics_demonstration.ipynb
locally, or through
binder.
from pprint import pprint
from functools import partial
import pandas as pd
from pandas.io.formats.style import Styler
from wombat.core import Simulation, Metrics
from wombat.utilities import plot
# Clean up the aesthetics for the pandas outputs
pd.set_option("display.max_rows", 30)
pd.set_option("display.max_columns", 10)
style = partial(
Styler,
table_attributes='style="font-size: 14px; grid-column-count: 6"',
precision=2,
thousands=",",
)
Table of Contents#
Below is a list of top-level sections to demonstrate how to use WOMBAT's Metrics
class methods and an explanation of each individual metric.
If you don't see a metric or result computation that is core to your work, please submit an issue with details on what the metric is, and how it should be computed.
Setup: Running a simulation to gather the results
Common Parameters: Explanation of frequently used parameter settings
Availability: Time-based and energy-based availability
Capacity Factor: Gross and net capacity factor
Task Completion Rate: Task completion metrics
Equipment Costs: Cost breakdowns by servicing equipment
Service Equipment Utilization Rate: Utilization of servicing equipment
Vessel-Crew Hours at Sea: Number of crew or vessel hours spent at sea
Number of Tows: Number of tows breakdowns
Labor Costs: Breakdown of labor costs
Equipment and Labor Costs: Combined servicing equipment and labor cost breakdown
Emissions: Emissions of servicing equipment based on activity
Component Costs: Materials costs
Fixed Cost Impacts: Total fixed costs
OpEx: Project OpEx
Process Times: Timing of various stages of repair and maintenance
Power Production: Potential and actually produced power
Net Present Value: Project NPV calculator
Setup#
The simulations from the How To notebook are going to be rerun as it is not recommended to create a Metrics class from scratch due to the large number of inputs that are required, and the initialization is provided in the simulation API's run method.
To simplify this process, a feature has been added to save the simulation outputs required to generate the Metrics inputs and a method to reload those outputs as inputs.
sim = Simulation("COREWIND", "morro_bay_in_situ.yaml")
# Both of these parameters are True by default for convenience
sim.run(create_metrics=True, save_metrics_inputs=True)
# Load the metrics data
fpath = sim.env.metrics_input_fname.parent
fname = sim.env.metrics_input_fname.name
metrics = Metrics.from_simulation_outputs(fpath, fname)
# Delete the log files now that they're loaded in
sim.env.cleanup_log_files()
# Alternatively, in this case because the simulation was run, we can use the
# following for convenience convenience only
metrics = sim.metrics
Common Parameter Explanations#
Before diving into each and every metric, and how they can be customized, it is worth noting some of the most common parameters used throughout, and their meanings to reduce redundancy. The varying output forms are demonstrated in the availability section below.
frequency
#
- project
Computed across the whole simulation, with the resulting
DataFrame
having an empty index.- annual
Summary of each year in the simulation, with the resulting
DataFrame
having "year" as the index.- monthly
Summary of each month of the year, aggregated across years, with the resulting
DataFrame
having "month" as the index.- month-year
computed on a month-by-year basis, producing the results for every month of the simulation, with the resulting
DataFrame
having "year" and "month" as the index.
by
#
- windfarm
Aggregated across all turbines, with the resulting
DataFrame
having only "windfarm" as a column- turbine
Computed for each turbine, with the resulting
DataFrame
having a column for each turbine
Availability#
There are two methods to produce availability, which have their own function calls:
energy: actual power produced divided by potential power produced
time: The ratio of all non-zero hours to all hours in the simulation, or the proportion of the simulation where turbines are operating
Here, we will go through the various input definitions to get time-based availability data as both methods use the same inputs, and provide outputs in the same format.
Inputs:
frequency
, as explained above options: "project", "annual", "monthly", and "month-year"by
, as explained above options: "windfarm" and "turbine"
Below is a demonstration of the variations on frequency
and by
for
time_based_availability
.
style(metrics.time_based_availability(frequency="project", by="windfarm"))
windfarm | |
---|---|
0 | 0.91 |
style(metrics.production_based_availability(frequency="project", by="windfarm"))
windfarm | |
---|---|
0 | 0.91 |
Note that in the two above examples, that the values are equal. This is due to the fact that the example simulation does not have any operating reduction applied to failures, unless it's a catastrophic failure, so there is no expected difference.
# Demonstrate the by turbine granularity
style(metrics.time_based_availability(frequency="project", by="turbine"))
WTG_0000 | WTG_0001 | WTG_0002 | WTG_0003 | WTG_0004 | WTG_0005 | WTG_0006 | WTG_0007 | WTG_0008 | WTG_0009 | WTG_0100 | WTG_0101 | WTG_0102 | WTG_0103 | WTG_0104 | WTG_0105 | WTG_0106 | WTG_0107 | WTG_0108 | WTG_0109 | WTG_0200 | WTG_0201 | WTG_0202 | WTG_0203 | WTG_0204 | WTG_0205 | WTG_0206 | WTG_0207 | WTG_0208 | WTG_0209 | WTG_0300 | WTG_0301 | WTG_0302 | WTG_0303 | WTG_0304 | WTG_0305 | WTG_0306 | WTG_0307 | WTG_0308 | WTG_0309 | WTG_0400 | WTG_0401 | WTG_0402 | WTG_0403 | WTG_0404 | WTG_0405 | WTG_0406 | WTG_0407 | WTG_0408 | WTG_0409 | WTG_0500 | WTG_0501 | WTG_0502 | WTG_0503 | WTG_0504 | WTG_0505 | WTG_0506 | WTG_0507 | WTG_0508 | WTG_0509 | WTG_0600 | WTG_0601 | WTG_0602 | WTG_0603 | WTG_0604 | WTG_0605 | WTG_0606 | WTG_0607 | WTG_0608 | WTG_0609 | WTG_0700 | WTG_0701 | WTG_0702 | WTG_0703 | WTG_0704 | WTG_0705 | WTG_0706 | WTG_0707 | WTG_0708 | WTG_0709 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 0.92 | 0.92 | 0.90 | 0.91 | 0.91 | 0.91 | 0.90 | 0.90 | 0.91 | 0.91 | 0.90 | 0.91 | 0.90 | 0.92 | 0.90 | 0.91 | 0.92 | 0.92 | 0.92 | 0.90 | 0.90 | 0.90 | 0.88 | 0.90 | 0.92 | 0.91 | 0.91 | 0.91 | 0.91 | 0.90 | 0.91 | 0.92 | 0.90 | 0.91 | 0.90 | 0.91 | 0.90 | 0.91 | 0.91 | 0.91 | 0.92 | 0.91 | 0.91 | 0.90 | 0.91 | 0.91 | 0.91 | 0.91 | 0.89 | 0.91 | 0.91 | 0.91 | 0.90 | 0.90 | 0.91 | 0.89 | 0.90 | 0.91 | 0.92 | 0.91 | 0.88 | 0.91 | 0.92 | 0.92 | 0.90 | 0.91 | 0.92 | 0.92 | 0.91 | 0.91 | 0.91 | 0.91 | 0.92 | 0.90 | 0.92 | 0.88 | 0.91 | 0.89 | 0.89 | 0.90 |
# Demonstrate the annualized outputs
style(metrics.time_based_availability(frequency="annual", by="windfarm"))
windfarm | |
---|---|
year | |
2002 | 0.95 |
2003 | 0.92 |
2004 | 0.92 |
2005 | 0.91 |
2006 | 0.88 |
2007 | 0.89 |
2008 | 0.89 |
2009 | 0.92 |
2010 | 0.89 |
2011 | 0.92 |
2012 | 0.89 |
2013 | 0.91 |
2014 | 0.92 |
2015 | 0.91 |
2016 | 0.89 |
2017 | 0.90 |
2018 | 0.91 |
2019 | 0.92 |
2020 | 0.93 |
2021 | 0.90 |
# Demonstrate the month aggregations
style(metrics.time_based_availability(frequency="monthly", by="windfarm"))
windfarm | |
---|---|
month | |
1 | 0.87 |
2 | 0.86 |
3 | 0.88 |
4 | 0.86 |
5 | 0.88 |
6 | 0.91 |
7 | 0.93 |
8 | 0.95 |
9 | 0.95 |
10 | 0.94 |
11 | 0.93 |
12 | 0.92 |
# Demonstrate the granular monthly reporting
style(metrics.time_based_availability(frequency="month-year", by="windfarm"))
windfarm | ||
---|---|---|
year | month | |
2002 | 1 | 0.94 |
2 | 0.93 | |
3 | 0.98 | |
4 | 0.98 | |
5 | 0.96 | |
6 | 0.95 | |
7 | 0.96 | |
8 | 0.98 | |
9 | 0.98 | |
10 | 0.96 | |
11 | 0.92 | |
12 | 0.91 | |
2003 | 1 | 0.85 |
2 | 0.90 | |
3 | 0.89 | |
4 | 0.89 | |
5 | 0.90 | |
6 | 0.89 | |
7 | 0.91 | |
8 | 0.96 | |
9 | 0.97 | |
10 | 0.94 | |
11 | 0.96 | |
12 | 0.93 | |
2004 | 1 | 0.87 |
2 | 0.90 | |
3 | 0.89 | |
4 | 0.89 | |
5 | 0.90 | |
6 | 0.90 | |
7 | 0.95 | |
8 | 0.97 | |
9 | 0.95 | |
10 | 0.97 | |
11 | 0.95 | |
12 | 0.93 | |
2005 | 1 | 0.62 |
2 | 0.92 | |
3 | 0.90 | |
4 | 0.90 | |
5 | 0.90 | |
6 | 0.91 | |
7 | 0.95 | |
8 | 0.98 | |
9 | 0.97 | |
10 | 0.94 | |
11 | 0.94 | |
12 | 0.95 | |
2006 | 1 | 0.70 |
2 | 0.61 | |
3 | 0.89 | |
4 | 0.90 | |
5 | 0.89 | |
6 | 0.90 | |
7 | 0.90 | |
8 | 0.91 | |
9 | 0.97 | |
10 | 0.97 | |
11 | 0.95 | |
12 | 0.94 | |
2007 | 1 | 0.83 |
2 | 0.67 | |
3 | 0.88 | |
4 | 0.91 | |
5 | 0.90 | |
6 | 0.90 | |
7 | 0.90 | |
8 | 0.90 | |
9 | 0.98 | |
10 | 0.95 | |
11 | 0.94 | |
12 | 0.92 | |
2008 | 1 | 0.84 |
2 | 0.62 | |
3 | 0.89 | |
4 | 0.92 | |
5 | 0.90 | |
6 | 0.89 | |
7 | 0.90 | |
8 | 0.90 | |
9 | 0.95 | |
10 | 0.94 | |
11 | 0.95 | |
12 | 0.93 | |
2009 | 1 | 0.93 |
2 | 0.83 | |
3 | 0.91 | |
4 | 0.92 | |
5 | 0.93 | |
6 | 0.93 | |
7 | 0.90 | |
8 | 0.94 | |
9 | 0.95 | |
10 | 0.93 | |
11 | 0.94 | |
12 | 0.96 | |
2010 | 1 | 0.92 |
2 | 0.90 | |
3 | 0.72 | |
4 | 0.71 | |
5 | 0.92 | |
6 | 0.92 | |
7 | 0.89 | |
8 | 0.95 | |
9 | 0.94 | |
10 | 0.93 | |
11 | 0.92 | |
12 | 0.93 | |
2011 | 1 | 0.93 |
2 | 0.90 | |
3 | 0.90 | |
4 | 0.86 | |
5 | 0.93 | |
6 | 0.94 | |
7 | 0.93 | |
8 | 0.91 | |
9 | 0.93 | |
10 | 0.92 | |
11 | 0.95 | |
12 | 0.93 | |
2012 | 1 | 0.91 |
2 | 0.90 | |
3 | 0.74 | |
4 | 0.67 | |
5 | 0.93 | |
6 | 0.94 | |
7 | 0.95 | |
8 | 0.94 | |
9 | 0.91 | |
10 | 0.94 | |
11 | 0.92 | |
12 | 0.89 | |
2013 | 1 | 0.90 |
2 | 0.89 | |
3 | 0.80 | |
4 | 0.78 | |
5 | 0.95 | |
6 | 0.95 | |
7 | 0.96 | |
8 | 0.97 | |
9 | 0.92 | |
10 | 0.90 | |
11 | 0.94 | |
12 | 0.94 | |
2014 | 1 | 0.93 |
2 | 0.93 | |
3 | 0.85 | |
4 | 0.92 | |
5 | 0.92 | |
6 | 0.90 | |
7 | 0.97 | |
8 | 0.96 | |
9 | 0.96 | |
10 | 0.91 | |
11 | 0.85 | |
12 | 0.89 | |
2015 | 1 | 0.93 |
2 | 0.94 | |
3 | 0.96 | |
4 | 0.78 | |
5 | 0.75 | |
6 | 0.97 | |
7 | 0.97 | |
8 | 0.98 | |
9 | 0.95 | |
10 | 0.94 | |
11 | 0.87 | |
12 | 0.89 | |
2016 | 1 | 0.82 |
2 | 0.90 | |
3 | 0.90 | |
4 | 0.76 | |
5 | 0.88 | |
6 | 0.90 | |
7 | 0.92 | |
8 | 0.96 | |
9 | 0.95 | |
10 | 0.93 | |
11 | 0.90 | |
12 | 0.89 | |
2017 | 1 | 0.89 |
2 | 0.89 | |
3 | 0.90 | |
4 | 0.72 | |
5 | 0.82 | |
6 | 0.90 | |
7 | 0.90 | |
8 | 0.96 | |
9 | 0.98 | |
10 | 0.95 | |
11 | 0.95 | |
12 | 0.92 | |
2018 | 1 | 0.91 |
2 | 0.90 | |
3 | 0.90 | |
4 | 0.91 | |
5 | 0.83 | |
6 | 0.90 | |
7 | 0.89 | |
8 | 0.96 | |
9 | 0.95 | |
10 | 0.95 | |
11 | 0.95 | |
12 | 0.92 | |
2019 | 1 | 0.89 |
2 | 0.89 | |
3 | 0.90 | |
4 | 0.89 | |
5 | 0.87 | |
6 | 0.87 | |
7 | 0.95 | |
8 | 0.97 | |
9 | 0.95 | |
10 | 0.93 | |
11 | 0.95 | |
12 | 0.91 | |
2020 | 1 | 0.90 |
2 | 0.90 | |
3 | 0.90 | |
4 | 0.91 | |
5 | 0.85 | |
6 | 0.94 | |
7 | 0.93 | |
8 | 0.98 | |
9 | 0.97 | |
10 | 0.97 | |
11 | 0.94 | |
12 | 0.90 | |
2021 | 1 | 0.89 |
2 | 0.91 | |
3 | 0.90 | |
4 | 0.89 | |
5 | 0.66 | |
6 | 0.87 | |
7 | 0.90 | |
8 | 0.96 | |
9 | 0.96 | |
10 | 0.97 | |
11 | 0.95 | |
12 | 0.96 |
Plotting Availability#
As of v0.9, the ability to plot the wind farm and turbine availability has been enabled as an experimental feature. Please see the plotting API documentation for more details.
# Demonstrate the granular monthly reporting
plot.plot_farm_availability(sim=sim, which="energy", farm_95_CI=True)
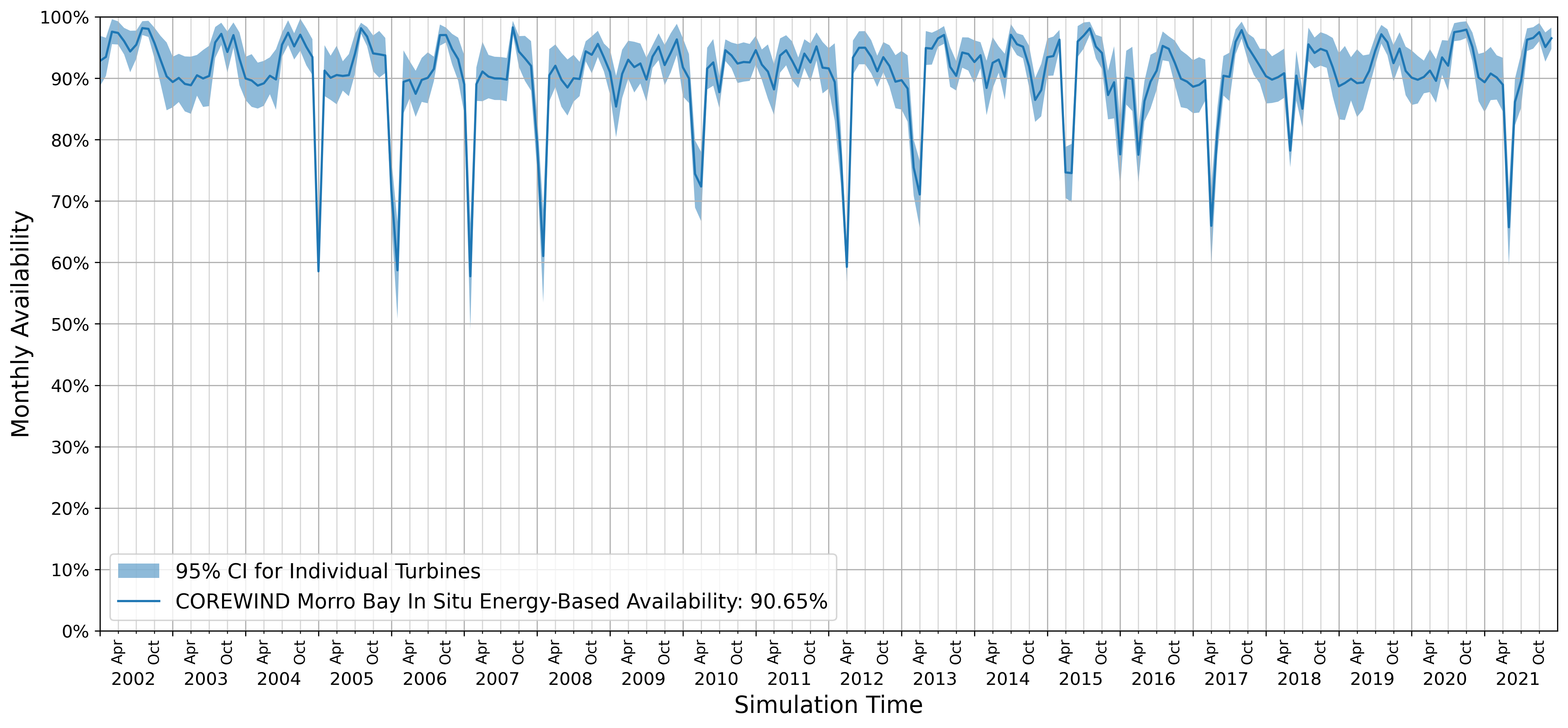
Capacity Factor#
The capacity factor is the ratio of actual (net) or potential (gross) energy production
divided by the project's capacity. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.capacity_factor()
.
Inputs:
which
"net": net capacity factor, actual production divided by the plant capacity
"gross": gross capacity factor, potential production divided by the plant capacity
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by
, as explained above, options: "windfarm" and "turbine"
Example Usage:
net_cf = metrics.capacity_factor(which="net", frequency="project", by="windfarm").values[0][0]
gross_cf = metrics.capacity_factor(which="gross", frequency="project", by="windfarm").values[0][0]
print(f" Net capacity factor: {net_cf:.2%}")
print(f"Gross capacity factor: {gross_cf:.2%}")
Net capacity factor: 50.40%
Gross capacity factor: 55.59%
Task Completion Rate#
The task completion rate is the ratio of tasks completed aggregated to the desired
frequency
. It is possible to have a >100% completion rate if all maintenance and
failure requests submitted in a time period were completed in addition to those that
went unfinished in prior time periods. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.task_completion_rate()
.
Inputs:
which
"scheduled": scheduled maintenance only (classified as maintenance tasks in inputs)
"unscheduled": unscheduled maintenance only (classified as failure events in inputs)
"both": Combined completion rate for all tasks
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"
Example Usage:
scheduled = metrics.task_completion_rate(which="scheduled", frequency="project").values[0][0]
unscheduled = metrics.task_completion_rate(which="unscheduled", frequency="project").values[0][0]
combined = metrics.task_completion_rate(which="both", frequency="project").values[0][0]
print(f" Scheduled Task Completion Rate: {scheduled:.2%}")
print(f"Unscheduled Task Completion Rate: {unscheduled:.2%}")
print(f" Overall Task Completion Rate: {combined:.2%}")
Scheduled Task Completion Rate: 96.43%
Unscheduled Task Completion Rate: 96.94%
Overall Task Completion Rate: 96.75%
Equipment Costs#
Sum of the costs associated with a simulation's servicing equipment, which excludes
materials, downtime, etc. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.equipment_costs()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_equipment
True
: Aggregates all equipment into a single costFalse
: Computes for each unit of servicing equipment
Example Usage:
# Project total at the whole wind farm level
style(metrics.equipment_costs(frequency="project", by_equipment=False))
equipment_cost | |
---|---|
0 | 2,329,868,795.11 |
# Project totals at servicing equipment level
style(metrics.equipment_costs(frequency="project", by_equipment=True))
Anchor Handling Tug | Cable Laying Vessel | Crew Transfer Vessel 1 | Crew Transfer Vessel 2 | Crew Transfer Vessel 3 | Crew Transfer Vessel 4 | Crew Transfer Vessel 5 | Crew Transfer Vessel 6 | Crew Transfer Vessel 7 | Diving Support Vessel | Heavy Lift Vessel | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | 130,064,593.21 | 168,440,914.04 | 25,563,183.10 | 25,563,206.29 | 25,563,219.69 | 25,563,224.62 | 25,563,210.43 | 25,563,205.93 | 25,563,201.22 | 145,177,374.89 | 1,707,243,461.68 |
Service Equipment Utilization Rate#
Ratio of days when the servicing equipment is in use (not delayed for a whole day due to
either weather or lack of repairs to be completed) to the number of days it's present in
the simulation. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.service_equipment_utilization()
.
Inputs:
frequency
, as explained above, options: "project" and "annual"
Example Usage:
# Project totals
style(metrics.service_equipment_utilization(frequency="project"))
Anchor Handling Tug | Cable Laying Vessel | Crew Transfer Vessel 1 | Crew Transfer Vessel 2 | Crew Transfer Vessel 3 | Crew Transfer Vessel 4 | Crew Transfer Vessel 5 | Crew Transfer Vessel 6 | Crew Transfer Vessel 7 | Diving Support Vessel | Heavy Lift Vessel | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | 0.84 | 0.91 | 1.00 | 1.00 | 1.00 | 1.00 | 1.00 | 1.00 | 1.00 | 0.89 | 0.97 |
Vessel-Crew Hours at Sea#
The number of vessel hours or crew hours at sea for offshore wind power plant
simulations. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.vessel_crew_hours_at_sea()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_equipment
True
: Aggregates all equipment into a single costFalse
: Computes for each unit of servicing equipment
vessel_crew_assumption
: A dictionary of vessel names (ServiceEquipment.settings.name
, but also found atMetrics.service_equipment_names
) and the number of crew onboard at any given time. The application of this assumption transforms the results from vessel hours at sea to crew hours at sea.
Example Usage:
# Project total, not broken out by vessel
style(metrics.vessel_crew_hours_at_sea(frequency="project", by_equipment=False))
Total Crew Hours at Sea | |
---|---|
0 | 699,633.07 |
# Annual project totals, broken out by vessel
style(metrics.vessel_crew_hours_at_sea(frequency="annual", by_equipment=True))
Total Crew Hours at Sea | Crew Transfer Vessel 1 | Crew Transfer Vessel 2 | Crew Transfer Vessel 3 | Crew Transfer Vessel 4 | Crew Transfer Vessel 5 | Crew Transfer Vessel 6 | Crew Transfer Vessel 7 | Heavy Lift Vessel | Cable Laying Vessel | Anchor Handling Tug | Diving Support Vessel | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
year | ||||||||||||
2002 | 20,033.19 | 1,211.20 | 1,770.61 | 1,266.12 | 1,533.39 | 1,707.70 | 1,659.53 | 1,543.35 | 6,130.43 | 934.26 | 2,276.60 | 0.00 |
2003 | 32,368.37 | 2,794.45 | 2,578.74 | 2,468.34 | 2,684.45 | 2,680.54 | 2,660.33 | 2,643.27 | 7,427.41 | 2,848.97 | 3,581.87 | 0.00 |
2004 | 37,389.63 | 2,393.32 | 2,517.61 | 2,447.23 | 2,384.63 | 2,467.35 | 2,416.31 | 2,432.32 | 7,844.78 | 4,625.11 | 1,822.00 | 6,038.97 |
2005 | 29,582.58 | 2,482.31 | 2,416.26 | 2,367.33 | 2,151.29 | 2,234.32 | 2,425.20 | 2,480.32 | 7,476.92 | 1,517.50 | 2,437.04 | 1,594.09 |
2006 | 36,250.54 | 2,606.72 | 2,520.16 | 2,554.04 | 2,790.73 | 2,829.17 | 2,561.99 | 2,730.24 | 7,441.73 | 2,503.84 | 1,702.34 | 6,009.58 |
2007 | 32,095.70 | 2,646.21 | 2,519.95 | 2,674.67 | 2,711.90 | 2,578.93 | 2,636.69 | 2,347.10 | 7,222.99 | 1,696.22 | 2,016.48 | 3,044.55 |
2008 | 39,430.28 | 2,576.30 | 2,742.56 | 2,776.01 | 2,583.23 | 2,567.65 | 2,668.00 | 2,535.12 | 7,406.13 | 2,392.40 | 5,115.51 | 6,067.37 |
2009 | 34,464.39 | 2,363.11 | 2,567.01 | 2,667.08 | 2,819.14 | 2,630.91 | 2,526.77 | 2,330.59 | 8,109.36 | 4,731.41 | 1,380.27 | 2,338.74 |
2010 | 33,666.30 | 2,660.85 | 2,446.38 | 2,573.21 | 2,660.17 | 2,794.65 | 2,643.73 | 2,659.14 | 6,983.51 | 809.66 | 2,244.12 | 5,190.86 |
2011 | 35,927.48 | 2,398.51 | 2,307.80 | 2,500.75 | 2,589.18 | 2,355.23 | 2,578.51 | 2,327.73 | 7,600.79 | 3,435.30 | 4,444.18 | 3,389.50 |
2012 | 37,857.87 | 2,670.02 | 2,534.60 | 2,706.36 | 2,456.51 | 2,525.40 | 2,510.93 | 2,674.90 | 7,042.84 | 4,412.28 | 3,719.68 | 4,604.35 |
2013 | 36,854.90 | 2,455.35 | 2,501.44 | 2,347.60 | 2,420.19 | 2,303.38 | 2,227.75 | 2,686.91 | 7,502.31 | 5,284.21 | 2,688.38 | 4,437.38 |
2014 | 34,786.33 | 2,255.12 | 2,376.96 | 2,458.61 | 2,399.81 | 2,352.55 | 2,281.19 | 2,557.49 | 8,121.38 | 3,396.43 | 1,723.76 | 4,863.04 |
2015 | 35,667.19 | 1,919.13 | 1,932.54 | 2,049.78 | 2,035.72 | 2,008.97 | 1,807.27 | 1,955.52 | 8,068.23 | 3,558.01 | 5,047.50 | 5,284.54 |
2016 | 38,079.48 | 2,664.61 | 2,728.87 | 2,769.65 | 2,628.09 | 2,597.55 | 2,559.64 | 2,818.92 | 7,605.12 | 3,985.86 | 3,160.31 | 4,560.86 |
2017 | 40,726.29 | 2,601.68 | 2,558.21 | 2,493.25 | 2,626.49 | 2,777.27 | 2,668.32 | 2,678.50 | 8,286.93 | 3,568.39 | 4,388.80 | 6,078.45 |
2018 | 33,684.29 | 2,244.52 | 2,346.38 | 2,257.16 | 2,309.02 | 2,247.72 | 2,220.66 | 2,469.96 | 7,666.44 | 1,035.81 | 4,799.95 | 4,086.67 |
2019 | 39,081.64 | 2,597.81 | 2,787.46 | 2,648.54 | 2,647.09 | 2,532.73 | 2,532.00 | 2,574.53 | 8,105.32 | 2,993.67 | 3,836.27 | 5,826.21 |
2020 | 32,162.34 | 2,290.09 | 2,561.75 | 2,490.59 | 2,158.53 | 2,221.42 | 2,458.08 | 2,377.83 | 7,435.30 | 2,094.10 | 2,073.38 | 4,001.29 |
2021 | 39,524.29 | 2,332.18 | 2,200.62 | 2,528.10 | 2,428.35 | 2,263.81 | 2,296.48 | 2,289.57 | 7,608.09 | 5,127.37 | 4,623.22 | 5,826.51 |
Number of Tows#
The number of tows performed during the simulation. If tow-to-port was not used in the
simulation, a DataFrame with a single value of 0 will be returned. For further
documentation, see the API docs here:
wombat.core.post_processor.Metrics.number_of_tows()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_tug
True
: Computed for each tugboat (towing vessel)False
: Aggregates all the tugboats
by_direction
True
: Computed for each direction a tow was performed (to port or to site)False
: Aggregates to the total number of tows
Example Usage:
# Project Total
# NOTE: This example has no towing, so it will return 0
style(metrics.number_of_tows(frequency="project"))
total_tows | |
---|---|
0 | 0 |
Labor Costs#
Sum of all labor costs associated with servicing equipment, excluding the labor defined
in the fixed costs, which can be broken out by type. For further documentation, see the
API docs here: wombat.core.post_processor.Metrics.labor_costs()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_type
True
: Computed for each labor type (salary and hourly)False
: Aggregates all the labor costs
Example Usage:
# Project total at the whole wind farm level
total = metrics.labor_costs(frequency="project", by_type=False)
print(f"Project total: ${total.values[0][0] / metrics.project_capacity:,.2f}/MW")
Project total: $42,253.78/MW
# Project totals for each type of labor
style(metrics.labor_costs(frequency="project", by_type=True))
hourly_labor_cost | salary_labor_cost | total_labor_cost | |
---|---|---|---|
0 | 0.00 | 50,704,531.67 | 50,704,531.67 |
Equipment and Labor Costs#
Sum of all labor and servicing equipment costs, excluding the labor defined in the fixed
costs, which can be broken out by each category. For further documentation, see the API
docs here: wombat.core.post_processor.Metrics.equipment_labor_cost_breakdown()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_category
True
: Computed for each unit servicing equipment and labor categoryFalse
: Aggregated to the sum of all costs
reason
definitions:
Maintenance: routine maintenance, or events defined as a
wombat.core.data_classes.Maintenance
Repair: unscheduled maintenance, ranging from inspections to replacements, or events defined as a
wombat.core.data_classes.Failure
Mobilization: Cost of mobilizing servicing equipment
Crew Transfer: Costs incurred while crew are transferring between a turbine or substation and the servicing equipment
Site Travel: Costs incurred while transiting to/from the site and while at the site
Weather Delay: Any delays caused by unsafe weather conditions
No Requests: Equipment and labor is active, but there are no repairs or maintenance tasks to be completed
Not in Shift: Any time outside the operating hours of the wind farm (or the servicing equipment's specific operating hours)
Example Usage:
# Project totals
style(metrics.equipment_labor_cost_breakdowns(frequency="project", by_category=False))
total_cost | total_hours | |
---|---|---|
reason | ||
Maintenance | 22,978,332.91 | 72,040.63 |
Repair | 601,365,747.80 | 166,151.09 |
Crew Transfer | 9,358,241.31 | 11,268.00 |
Site Travel | 100,566,232.83 | 125,041.05 |
Mobilization | 136,245,000.00 | 153,432.00 |
Weather Delay | 1,390,306,320.38 | 611,918.40 |
No Requests | 91,160,337.77 | 388,895.06 |
Not in Shift | 28,593,113.78 | 107,629.72 |
# Project totals by each category
style(metrics.equipment_labor_cost_breakdowns(frequency="project", by_category=True))
hourly_labor_cost | salary_labor_cost | total_labor_cost | equipment_cost | total_cost | total_hours | |
---|---|---|---|---|---|---|
reason | ||||||
Maintenance | 0 | 2,194,282.98 | 2,194,282.98 | 20,784,049.92 | 22,978,332.91 | 72,040.63 |
Repair | 0 | 7,929,579.52 | 7,929,579.52 | 593,436,168.29 | 601,365,747.80 | 166,151.09 |
Crew Transfer | 0 | 352,309.02 | 352,309.02 | 9,005,932.29 | 9,358,241.31 | 11,268.00 |
Site Travel | 0 | 3,946,967.80 | 3,946,967.80 | 96,619,265.03 | 100,566,232.83 | 125,041.05 |
Mobilization | 0 | 0.00 | 0.00 | 136,245,000.00 | 136,245,000.00 | 153,432.00 |
Weather Delay | 0 | 22,124,430.75 | 22,124,430.75 | 1,368,181,889.63 | 1,390,306,320.38 | 611,918.40 |
No Requests | 0 | 11,065,800.02 | 11,065,800.02 | 80,094,537.75 | 91,160,337.77 | 388,895.06 |
Not in Shift | 0 | 3,091,161.59 | 3,091,161.59 | 25,501,952.19 | 28,593,113.78 | 107,629.72 |
Emissions#
Emissions (tons or other provided units) of all servicing equipment activity, except
overnight waiting periods between shifts. For further documentation, see the API docs
here: wombat.core.post_processor.Metrics.emissions()
.
Inputs:
emissions_factors
: Dictionary of servicing equipment names and the emissions per hour of the following activities:transit
,maneuvering
,idle at site
, andidle at port
, where port is stand-in for wherever the servicing equipment might be based when not at site.maneuvering_factor
: The proportion of transit time that can generally be associated with positioning servicing, by default 10%.port_engine_on_factor
: The proportion of the idling at port time when the engine is running and producing emissions, by default 25%.
# Create the emissions factors, in tons per hour
emissions_factors = {
"Crew Transfer Vessel 1": {
"transit": 4,
"maneuvering": 3,
"idle at site": 0.5,
"idle at port": 0.25,
},
"Field Support Vessel": {
"transit": 6,
"maneuvering": 4,
"idle at site": 1,
"idle at port": 0.5,
},
"Heavy Lift Vessel": {
"transit": 12,
"maneuvering": 7,
"idle at site": 1,
"idle at port": 0.5,
},
"Diving Support Vessel": {
"transit": 4,
"maneuvering": 7,
"idle at site": 0.2,
"idle at port": 0.2,
},
"Anchor Handling Vessel": {
"transit": 4,
"maneuvering": 3,
"idle at site": 1,
"idle at port": 0.25,
},
}
# Add in CTVs 2 through 7
for i in range(2, 8):
emissions_factors[f"Crew Transfer Vessel {i}"] = emissions_factors[f"Crew Transfer Vessel 1"]
style(metrics.emissions(emissions_factors=emissions_factors, maneuvering_factor=0.075, port_engine_on_factor=0.20))
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
Cell In[22], line 39
36 for i in range(2, 8):
37 emissions_factors[f"Crew Transfer Vessel {i}"] = emissions_factors[f"Crew Transfer Vessel 1"]
---> 39 style(metrics.emissions(emissions_factors=emissions_factors, maneuvering_factor=0.075, port_engine_on_factor=0.20))
File /opt/hostedtoolcache/Python/3.11.12/x64/lib/python3.11/site-packages/wombat/core/post_processor.py:1623, in Metrics.emissions(self, emissions_factors, maneuvering_factor, port_engine_on_factor)
1588 """Calculates the emissions, typically in tons, per hour of operations for
1589 transiting, maneuvering (calculated as a % of transiting), idling at the site
1590 (repairs, crew transfer, weather delays), and idling at port (weather delays),
(...) 1618 equipment definition in ``emissions_factors``.
1619 """
1620 if missing := set(self.service_equipment_names).difference(
1621 [*emissions_factors]
1622 ):
-> 1623 raise KeyError(
1624 f"`emissions_factors` is missing the following keys: {missing}"
1625 )
1627 valid_categories = ("transit", "maneuvering", "idle at port", "idle at site")
1628 emissions_categories = list(
1629 chain(*[[*val] for val in emissions_factors.values()])
1630 )
KeyError: "`emissions_factors` is missing the following keys: {'Cable Laying Vessel', 'Anchor Handling Tug'}"
Component Costs#
All the costs associated with maintenance and failure events during the simulation,
including delays incurred during the repair process, but excluding costs not directly
tied to a repair. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.component_costs()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_category
True
: Computed across each cost categoryFalse
: Aggregated to the sum of all categories
by_action
True
: Computed by each of "repair", "maintenance", and "delay", and is included in the MultiIndexFalse
: Aggregated as the sum of all actions
action
definitions:
maintenance: routine maintenance
repair: unscheduled maintenance, ranging from inspections to replacements
delay: Any delays caused by unsafe weather conditions or not being able to finish a process within a single shift
Example Usage:
# Project totals by component
style(metrics.component_costs(frequency="project", by_category=False, by_action=False))
total_cost | |
---|---|
component | |
cable | 174,220,599.17 |
drive_train | 172,256,150.29 |
electrical_system | 1,212,816,670.16 |
generator | 271,214,580.38 |
hydraulic_system | 32,677,491.28 |
rotor_blades | 92,315,763.69 |
supporting_structure | 234,513,540.97 |
transformer | 1,305,211.33 |
yaw_system | 15,373,925.82 |
# Project totals by each category and action type
style(metrics.component_costs(frequency="project", by_category=True, by_action=True))
materials_cost | total_labor_cost | equipment_cost | total_cost | ||
---|---|---|---|---|---|
component | action | ||||
cable | delay | 0.00 | 2,857,398.66 | 97,775,754.82 | 100,633,153.48 |
maintenance | 7,000.00 | 15,342.60 | 525,000.00 | 547,342.60 | |
repair | 7,240,000.00 | 1,753,404.28 | 59,998,777.78 | 68,992,182.06 | |
drive_train | delay | 0.00 | 900,607.46 | 115,113,541.96 | 116,014,149.42 |
maintenance | 0.00 | 0.00 | 0.00 | 0.00 | |
repair | 4,965,000.00 | 268,209.70 | 45,754,580.09 | 50,987,789.80 | |
electrical_system | delay | 0.00 | 5,014,634.65 | 820,451,778.95 | 825,466,413.60 |
maintenance | 0.00 | 0.00 | 0.00 | 0.00 | |
repair | 9,228,000.00 | 1,678,612.01 | 323,854,233.45 | 334,760,845.46 | |
generator | delay | 0.00 | 4,764,942.22 | 180,555,868.10 | 185,320,810.32 |
maintenance | 2,146,500.00 | 940,939.74 | 5,008,500.00 | 8,095,939.74 | |
repair | 3,882,060.00 | 500,651.29 | 59,246,833.10 | 63,629,544.39 | |
hydraulic_system | delay | 0.00 | 3,409,760.08 | 18,149,709.95 | 21,559,470.03 |
maintenance | 0.00 | 0.00 | 0.00 | 0.00 | |
repair | 1,088,200.00 | 861,706.17 | 4,586,750.00 | 6,536,656.17 | |
rotor_blades | delay | 0.00 | 1,651,334.26 | 50,671,191.92 | 52,322,526.17 |
maintenance | 0.00 | 0.00 | 0.00 | 0.00 | |
repair | 6,073,980.00 | 455,346.45 | 30,343,125.00 | 36,872,451.45 | |
supporting_structure | delay | 0.00 | 6,157,011.74 | 102,434,169.65 | 108,591,181.40 |
maintenance | 1,106,300.00 | 1,213,014.12 | 15,117,549.92 | 17,436,864.05 | |
repair | 17,609,500.00 | 2,324,364.96 | 63,987,994.10 | 83,921,859.06 | |
transformer | delay | 0.00 | 141,470.09 | 753,026.92 | 894,497.02 |
maintenance | 19,000.00 | 24,986.52 | 133,000.00 | 176,986.52 | |
repair | 24,000.00 | 3,945.24 | 21,000.00 | 48,945.24 | |
yaw_system | delay | 0.00 | 317,943.53 | 7,776,193.27 | 8,094,136.80 |
maintenance | 0.00 | 0.00 | 0.00 | 0.00 | |
repair | 139,500.00 | 83,339.42 | 5,642,874.77 | 5,865,714.18 |
Fixed Cost Impacts#
Computes the total costs of the fixed costs categories. For further documentation, see
the definition docs, here: wombat.core.data_classes.FixedCosts
, or the API
docs here: wombat.core.post_processor.Metrics.fixed_costs()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"resolution
(also, demonstrated below)"high": Computed across the most granular cost levels
"medium": Computed for each general cost category
"low": Aggregated to a single sum of costs
pprint(metrics.fixed_costs.hierarchy)
{'operations': {'annual_leases_fees': ['submerge_land_lease_costs',
'transmission_charges_rights'],
'environmental_health_safety_monitoring': [],
'insurance': ['brokers_fee',
'operations_all_risk',
'business_interruption',
'third_party_liability',
'storm_coverage'],
'labor': [],
'onshore_electrical_maintenance': [],
'operating_facilities': [],
'operations_management_administration': ['project_management_administration',
'marine_management',
'weather_forecasting',
'condition_monitoring']}}
Example Usage:
# Project totals at the highest level
# NOTE: there were no fixed costs defined in this example, so all values will be 0, so
# this will just be demonstrating the output format
style(metrics.project_fixed_costs(frequency="project", resolution="low"))
operations | |
---|---|
0 | 0.00 |
# Project totals at the medium level
style(metrics.project_fixed_costs(frequency="project", resolution="medium"))
operations_management_administration | insurance | annual_leases_fees | operating_facilities | environmental_health_safety_monitoring | onshore_electrical_maintenance | labor | |
---|---|---|---|---|---|---|---|
0 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 |
# Project totals at the lowest level
style(metrics.project_fixed_costs(frequency="project", resolution="high"))
project_management_administration | marine_management | weather_forecasting | condition_monitoring | brokers_fee | operations_all_risk | business_interruption | third_party_liability | storm_coverage | submerge_land_lease_costs | transmission_charges_rights | operating_facilities | environmental_health_safety_monitoring | onshore_electrical_maintenance | labor | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 | 0.00 |
OpEx#
Computes the total cost of all operating expenditures for the duration of the
simulation, including fixed costs. For further documentation, see the API docs here:
wombat.core.post_processor.Metrics.opex()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by_category
True
shows the port fees, fixed costs, labor costs, equipment costs, and materials costs in addition the total OpExFalse
shows only the total OpEx
Example Usage:
style(metrics.opex("annual"))
/opt/hostedtoolcache/Python/3.11.12/x64/lib/python3.11/site-packages/wombat/core/post_processor.py:2054: FutureWarning: Downcasting object dtype arrays on .fillna, .ffill, .bfill is deprecated and will change in a future version. Call result.infer_objects(copy=False) instead. To opt-in to the future behavior, set `pd.set_option('future.no_silent_downcasting', True)`
port_fees = port_fees.fillna(0)
OpEx | |
---|---|
year | |
2002 | 92,079,422.57 |
2003 | 119,343,997.79 |
2004 | 133,656,817.55 |
2005 | 105,138,863.67 |
2006 | 125,113,221.06 |
2007 | 111,552,176.45 |
2008 | 127,950,522.32 |
2009 | 123,036,098.16 |
2010 | 117,730,122.84 |
2011 | 123,429,308.83 |
2012 | 128,699,340.30 |
2013 | 126,400,813.90 |
2014 | 120,228,276.05 |
2015 | 124,995,858.37 |
2016 | 129,137,842.52 |
2017 | 127,980,769.23 |
2018 | 126,386,460.17 |
2019 | 128,925,156.02 |
2020 | 111,163,042.74 |
2021 | 131,154,256.24 |
style(metrics.opex("annual", by_category=True))
/opt/hostedtoolcache/Python/3.11.12/x64/lib/python3.11/site-packages/wombat/core/post_processor.py:2054: FutureWarning: Downcasting object dtype arrays on .fillna, .ffill, .bfill is deprecated and will change in a future version. Call result.infer_objects(copy=False) instead. To opt-in to the future behavior, set `pd.set_option('future.no_silent_downcasting', True)`
port_fees = port_fees.fillna(0)
operations | port_fees | equipment_cost | total_labor_cost | materials_cost | OpEx | |
---|---|---|---|---|---|---|
year | ||||||
2002 | 0.00 | 0 | 88,411,309.36 | 2,123,473.21 | 1,544,640.00 | 92,079,422.57 |
2003 | 0.00 | 0 | 114,593,710.60 | 2,404,487.18 | 2,345,800.00 | 119,343,997.79 |
2004 | 0.00 | 0 | 128,144,211.69 | 2,649,305.87 | 2,863,300.00 | 133,656,817.55 |
2005 | 0.00 | 0 | 99,480,849.93 | 2,226,093.74 | 3,431,920.00 | 105,138,863.67 |
2006 | 0.00 | 0 | 119,922,283.48 | 2,532,333.59 | 2,658,604.00 | 125,113,221.06 |
2007 | 0.00 | 0 | 107,294,403.38 | 2,270,301.07 | 1,987,472.00 | 111,552,176.45 |
2008 | 0.00 | 0 | 123,254,999.12 | 2,661,619.20 | 2,033,904.00 | 127,950,522.32 |
2009 | 0.00 | 0 | 117,087,627.43 | 2,547,398.73 | 3,401,072.00 | 123,036,098.16 |
2010 | 0.00 | 0 | 113,547,495.87 | 2,392,626.97 | 1,790,000.00 | 117,730,122.84 |
2011 | 0.00 | 0 | 117,491,841.03 | 2,620,987.81 | 3,316,480.00 | 123,429,308.83 |
2012 | 0.00 | 0 | 123,173,019.19 | 2,737,457.10 | 2,788,864.00 | 128,699,340.30 |
2013 | 0.00 | 0 | 120,637,151.23 | 2,726,322.67 | 3,037,340.00 | 126,400,813.90 |
2014 | 0.00 | 0 | 115,394,074.49 | 2,460,525.57 | 2,373,676.00 | 120,228,276.05 |
2015 | 0.00 | 0 | 118,502,637.37 | 2,659,589.00 | 3,833,632.00 | 124,995,858.37 |
2016 | 0.00 | 0 | 124,672,731.77 | 2,683,610.75 | 1,781,500.00 | 129,137,842.52 |
2017 | 0.00 | 0 | 121,948,234.13 | 2,727,955.09 | 3,304,580.00 | 127,980,769.23 |
2018 | 0.00 | 0 | 120,834,983.22 | 2,486,176.95 | 3,065,300.00 | 126,386,460.17 |
2019 | 0.00 | 0 | 122,899,987.51 | 2,637,136.51 | 3,388,032.00 | 128,925,156.02 |
2020 | 0.00 | 0 | 106,703,938.68 | 2,375,572.06 | 2,083,532.00 | 111,163,042.74 |
2021 | 0.00 | 0 | 125,873,305.64 | 2,781,558.60 | 2,499,392.00 | 131,154,256.24 |
Process Times#
Computes the total number of hours spent from repair request submission to completion,
performing repairs, and the number of request for each repair category. For further
documentation, see the API docs here:
wombat.core.post_processor.Metrics.process_times()
.
Example Usage:
style(metrics.process_times())
time_to_completion | process_time | downtime | time_to_start | N | |
---|---|---|---|---|---|
category | |||||
anchor replacement | 15,581.89 | 8,709.37 | 8,708.14 | 7,277.41 | 18 |
annual turbine inspection | 1,463,749.36 | 168,264.46 | 166,806.16 | 1,306,482.37 | 1,432 |
array cable major repair | 42,967.68 | 26,177.25 | 0.00 | 16,172.01 | 41 |
array cable replacement | 34,409.58 | 23,958.77 | 0.00 | 11,581.14 | 30 |
blades major repair | 185,401.18 | 1,283.86 | 1,276.40 | 184,185.43 | 19 |
blades major replacement | 6,150.97 | 4,705.19 | 4,705.19 | 1,536.02 | 2 |
blades minor repair | 146,403.55 | 78,511.36 | 77,928.76 | 80,458.76 | 705 |
buoyancy module replacement | 12,388.08 | 10,508.11 | 10,463.97 | 3,165.61 | 46 |
direct drive generator major repair | 442,414.44 | 7,409.77 | 7,364.82 | 414,412.10 | 48 |
direct drive generator major replacement | 18,711.84 | 11,162.80 | 11,158.68 | 7,873.32 | 11 |
direct drive generator minor repair | 122,482.41 | 62,175.91 | 61,516.24 | 75,308.60 | 793 |
export cable major repair | 0.00 | 0.00 | 0.00 | 0.00 | 1 |
export cable subsea inspection | 7,678.94 | 1,778.39 | 0.00 | 6,539.49 | 20 |
main shaft major repair | 355,697.21 | 4,096.18 | 4,085.50 | 351,778.66 | 41 |
main shaft minor repair | 60,865.79 | 24,442.69 | 24,125.96 | 42,209.96 | 355 |
main shaft replacement | 21,018.90 | 9,347.39 | 9,339.97 | 12,508.24 | 18 |
major anchor repair | 19,241.41 | 8,321.22 | 8,320.08 | 11,077.91 | 27 |
major pitch system repair | 69,125.97 | 53,697.83 | 53,518.51 | 18,853.39 | 243 |
marine growth removal | 54,654.17 | 36,198.02 | 36,048.80 | 21,729.31 | 175 |
minor ballast pump repair | 1,009.90 | 501.22 | 495.70 | 536.35 | 12 |
minor pitch system repair | 239,681.04 | 128,154.70 | 127,039.57 | 132,955.32 | 1,230 |
mooring line major repair | 18,163.07 | 7,873.20 | 7,872.04 | 10,537.58 | 21 |
mooring line replacement | 19,305.87 | 10,469.93 | 10,466.87 | 9,136.09 | 18 |
oss annual inspection | 12,330.24 | 6,698.49 | 6,660.45 | 6,670.84 | 38 |
oss minor repair | 1,871.21 | 587.81 | 571.67 | 1,435.93 | 12 |
power converter major repair | 3,646,369.77 | 32,802.36 | 32,691.59 | 3,394,974.60 | 468 |
power converter minor repair | 142,079.96 | 68,904.61 | 68,131.34 | 86,599.32 | 813 |
power converter replacement | 169,353.62 | 60,379.65 | 60,349.75 | 112,822.84 | 100 |
power electrical system major repair | 263,994.05 | 2,646.56 | 2,639.79 | 231,106.00 | 32 |
power electrical system major replacement | 4,453.30 | 1,929.68 | 1,928.85 | 3,205.85 | 4 |
power electrical system minor repair | 86,821.68 | 34,305.96 | 33,859.99 | 61,043.40 | 560 |
structural annual inspection | 1,390,183.85 | 156,594.78 | 155,340.66 | 1,239,107.12 | 1,395 |
structural subsea inspection | 687,222.99 | 24,895.34 | 24,330.93 | 672,003.87 | 668 |
yaw system major repair | 75,902.39 | 1,037.65 | 1,034.29 | 74,945.63 | 10 |
yaw system minor repair | 37,091.45 | 15,290.54 | 15,089.68 | 25,413.25 | 231 |
Power Production#
Computes the total power production for the wind farm. For further documentation, see
the API docs here: wombat.core.post_processor.Metrics.power_production()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"by
, as explained above options: "windfarm" and "turbine"units
"kwh": kilowatt-hours (kWh)
"mwh": megawatt-hours (MWh)
"gwh": gigawatt-hours (GWh)
Example Usage:
# Project totals, in kWh, at the wind farm level
style(metrics.power_production(frequency="project", by="windfarm", units="kwh"))
windfarm | |
---|---|
Project Energy Production (kWh) | 106,027,078,988.00 |
# Project totals, in MWh, at the wind farm level
style(metrics.power_production(frequency="project", units="mwh"))
windfarm | |
---|---|
Project Energy Production (MWh) | 106,027,078.99 |
# Project totals, in GWh, at the wind farm level
style(metrics.power_production(frequency="project"))
windfarm | |
---|---|
Project Energy Production (GWh) | 106,027.08 |
Net Present Value#
Calcualtes the net present value (NPV) for the project, as \(NPV = (Power * OfftakePrice - OpEx) / (1 + DiscountRate)\).
For further documentation, see the API docs here: wombat.core.post_processor.Metrics.npv()
.
Inputs:
frequency
, as explained above, options: "project", "annual", "monthly", and "month-year"discount_rate
: The rate of return that could be earned on alternative investments, by default 0.025.offtake_price
: Price of energy, per MWh, by default 80.
style(metrics.opex("annual"))
/opt/hostedtoolcache/Python/3.11.12/x64/lib/python3.11/site-packages/wombat/core/post_processor.py:2054: FutureWarning: Downcasting object dtype arrays on .fillna, .ffill, .bfill is deprecated and will change in a future version. Call result.infer_objects(copy=False) instead. To opt-in to the future behavior, set `pd.set_option('future.no_silent_downcasting', True)`
port_fees = port_fees.fillna(0)
OpEx | |
---|---|
year | |
2002 | 92,079,422.57 |
2003 | 119,343,997.79 |
2004 | 133,656,817.55 |
2005 | 105,138,863.67 |
2006 | 125,113,221.06 |
2007 | 111,552,176.45 |
2008 | 127,950,522.32 |
2009 | 123,036,098.16 |
2010 | 117,730,122.84 |
2011 | 123,429,308.83 |
2012 | 128,699,340.30 |
2013 | 126,400,813.90 |
2014 | 120,228,276.05 |
2015 | 124,995,858.37 |
2016 | 129,137,842.52 |
2017 | 127,980,769.23 |
2018 | 126,386,460.17 |
2019 | 128,925,156.02 |
2020 | 111,163,042.74 |
2021 | 131,154,256.24 |